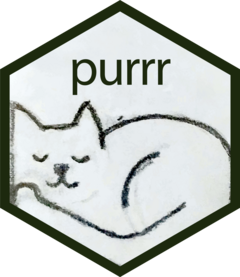
Package index
Map family
The map(.x, .f)
functions transforms each element of the vector .x
with the function .f
, returning a vector defined by the suffix (_lgl
, _chr()
etc). walk()
is a variant for functions called primarily for their side-effects; it returns .x
invisibly.
As well as functions, .f
, can take numbers and characters (used as a shorthand for [[
), and formulas (used as a succint function definition). See details in as_mapper()
-
map()
map_lgl()
map_int()
map_dbl()
map_chr()
map_vec()
walk()
- Apply a function to each element of a vector
-
as_mapper()
- Convert an object into a mapper function
Map variants
A rich set of variants builds on the basic idea of map()
: modify()
modifies “in place”, returning a vector the same type as .x
; map2()
iterates over two vectors in parallel; pmap()
(parallel map) iterates over a list of vectors; imap()
(index map) is a shortcut for the common pattern map2(x, names(x))
.
-
map_depth()
modify_depth()
- Map/modify elements at given depth
-
map2()
map2_lgl()
map2_int()
map2_dbl()
map2_chr()
map2_vec()
walk2()
- Map over two inputs
-
pmap()
pmap_lgl()
pmap_int()
pmap_dbl()
pmap_chr()
pmap_vec()
pwalk()
- Map over multiple input simultaneously (in "parallel")
-
modify()
modify_if()
modify_at()
modify2()
imodify()
- Modify elements selectively
-
modify_tree()
- Recursively modify a list
-
imap()
imap_lgl()
imap_chr()
imap_int()
imap_dbl()
imap_vec()
iwalk()
- Apply a function to each element of a vector, and its index
Predicate functionals
A predicate function is a function that either returns TRUE
or FALSE
. The predicate functionals take a vector and a predicate function and do something useful.
-
detect()
detect_index()
- Find the value or position of the first match
-
has_element()
- Does a list contain an object?
-
head_while()
tail_while()
- Find head/tail that all satisfies a predicate.
-
keep_at()
discard_at()
- Keep/discard elements based on their name/position
-
pluck()
`pluck<-`()
pluck_exists()
- Safely get or set an element deep within a nested data structure
-
chuck()
- Get an element deep within a nested data structure, failing if it doesn't exist
-
pluck_depth()
- Compute the depth of a vector
-
modify_in()
assign_in()
- Modify a pluck location
-
attr_getter()
- Create an attribute getter function
-
accumulate()
accumulate2()
- Accumulate intermediate results of a vector reduction
-
list_c()
list_cbind()
list_rbind()
- Combine list elements into a single data structure
-
list_flatten()
- Flatten a list
-
list_assign()
list_modify()
list_merge()
- Modify a list
-
list_simplify()
- Simplify a list to an atomic or S3 vector
-
list_transpose()
- Transpose a list
Superseded
Superseded functions have been replaced by superior solutions, but due to their widespread use will not go away. However, they will not get any new features and will only receive critical bug fixes.
-
flatten()
flatten_lgl()
flatten_int()
flatten_dbl()
flatten_chr()
flatten_dfr()
flatten_dfc()
superseded - Flatten a list of lists into a simple vector
-
map_dfr()
map_dfc()
imap_dfr()
imap_dfc()
map2_dfr()
map2_dfc()
pmap_dfr()
pmap_dfc()
superseded - Functions that return data frames
-
as_vector()
simplify()
simplify_all()
superseded - Coerce a list to a vector
-
transpose()
superseded - Transpose a list.
Adverbs
Adverbs modify the action of a function; they take a function as input and return a function with modified action as output.
-
auto_browse()
- Wrap a function so it will automatically
browse()
on error
-
compose()
- Compose multiple functions together to create a new function
-
insistently()
- Transform a function to wait then retry after an error
-
negate()
- Negate a predicate function so it selects what it previously rejected
-
partial()
- Partially apply a function, filling in some arguments
-
possibly()
- Wrap a function to return a value instead of an error
-
quietly()
- Wrap a function to capture side-effects
-
safely()
- Wrap a function to capture errors
-
slowly()
- Wrap a function to wait between executions
-
array_branch()
array_tree()
- Coerce array to list
-
rate_delay()
rate_backoff()
is_rate()
- Create delaying rate settings
-
progress_bars
- Progress bars in purrr
-
in_parallel()
experimental - Parallelization in purrr